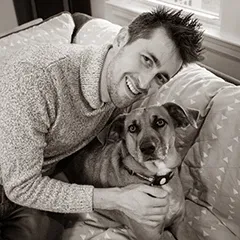
Location Boston, MA
Interests Programming, Photography, Cooking, Game Development, Reading, Guitar, Running, Travel, Never having enough time...
Working On fusion power at CFS
This Site Made with Astro, Cloudinary , and many many hours of writing, styling, editing, breaking things , fixing things, and hoping it all works out.
Education B.S. Computer Science, New Mexico Tech
Contact site at dillonshook dot com
Random Read another random indie blog on the interweb
Subscribe Get the inside scoop and $10 off any print!
The Pseudocode Programming Process
Today I’m going to walk you through the Pseudocode Programming Process and how you can use it in a couple different scenarios to streamline your process. I first read about this technique in the book Code Complete 2 when I first read it circa 2008 (has it really been that long?) but came back to it again not too long ago. The book has held up quite well despite being over 20 years old and I would still strongly recommend it to anyone early to mid level in their programming career. You can still find a number of good gems if you’re more experienced than that but you’ll also probably be skimming quite a lot since it’s quite the tome.
The book dedicates an entire chapter to the Pseudocode Programming Process (PPP from here out) so I’ll be summarizing it here and giving it a fresh look from the very different world we live in 20 years after this was written.
What is PPP?
Simply put, the Pseudocode Programming Process is a structured methodology for writing a class or function first in pseudocode, iterating on the pseudocode, then completing the function by converting the pseudocode into code.
But what is pseudocode exactly?
Pseudocode is English-like (or whatever human language you think best in) statements that precisely describe the operations the computer will take. It doesn’t include any syntax or language specific idioms or operations. Instead, it’s describing at a higher level the intent of each step in the function. But it’s not written at so high of a level that you’ll gloss over problems when writing the code. It should be fairly obvious what the code looks like that satisfies each line of pseudocode. Let’s get some examples though since those are generally more helpful than descriptions.
Examples
Bad PseudocodeCreate SagaResource and start polling status await status complete catch(error){ log error and show error }
This is bad psuedocode because it’s both too high level and misses a number of important details, as well as being too low level with language specific
catch(error)
syntax. It’s hard to fully understand what the block will do or what all the steps will be when it’s fleshed out.
Good PseudocodeSend request to create SagaResource If request was successful Begin polling SagaResourceStatus If status is still in progress Keep polling If status is failed Stop polling Log error Set error state with retry message to show the user If status is success Stop polling Send request to get SagaResourceDetails If request fails Retry twice If all still failed Set error state with error message to user Else if succeeded Set success state and show success message to user Else Log error Set error state with error message to show the user
This pseudocode is much better since it stays at a consistent level of intent with each statement without being too low level. It’s easy to follow the flow and understand what sort of code will be produced by turning it into a working function.
The Process
Now that we have a good idea of what pseudocode is lets talk about the Process in the Pseudocode Programming Process.
1. Design the function
Start by defining the problem the method will solve, then methodically think through all the important factors a method needs to consider:
- What are the prerequisites/preconditions that must be true before calling the function?
- What is the input and output type signature?
- What are the postconditions that the function will guaranteed to be true after it returns?
- What will the name be?
- How will you test the function?
- How will errors be handled?
- What will the performance characteristics be?
- Will 3rd party libraries be needed?
- What other dependencies are there on the rest of the system?
- What algorithms and data types are most suited to the problem?
2. Write the function using pseudocode
Now with a good idea of how the method should work start writing the pseudocode in your editor. Start with the header comment describing what the purpose of the function is as well as the pre & post conditions. If it’s hard to summarize, take that as a sign you need to spend more time on the design and clarify its intent.
Next write the function name, input parameters, and final return statement as the skeleton. Proceed with writing the rest of the pseudocode statements to fill out the function. If you’re working on a particularly complex function take the time to try a couple of different approaches in pseudocode before settling on the best one. The best time to change something is the earliest time when you’re the least invested in the code.
3. Check the pseudocode
Take a step back now and try to look at your pseudocode with a fresh mind of someone who’s not familiar with the problem. Does everything make sense? Can you spot any bugs now? If the function is particularly tricky now’s a good time to get a review from a coworker. Pseudocode is much easier and quicker to review than a full PR so don’t be afraid to ask for an early review to see if you missed any assumptions or alternatives.
4. Code the function
You should now be ready to implement the function from the pseudocode following a simple procedure:
- Start by writing the function signature and final statements, this time in real code.
- Turn the pseudocode into line level comments
- Fill in the code below each pseudocode comment
- Check the code, refactoring as necessary or applying the PPP recursively if one statement needs to be turned into its own function
- Clean up any leftovers and comments that are unhelpfully obvious
Benefits
The process is a bit more involved than slapping together a function like you might be used to so why bother?
Here’s a few reasons:
- Have you ever gotten stuck in the weeds in the middle of writing a function or component and lost your train of thought? PPP lets you stay high level and design the structure before worring about the details.
- Have you ever forget to check a pre or post condition while writing code? Being more intentional with your process using PPP helps you think through all the different dimensions a function needs to consider.
- Have ever discovered a design flaw while in the middle of writing a function? Writing the code first with PPP helps you see errors and design flaws early in the process when they’re the cheapest to fix and easiest to iterate on.
- Have you ever forgotten to document your code? With PPP you’re writing good comments first so your code will always be documented!
AI Level Up
Here’s one of the best parts that Steve McConnell probably didn’t envision when he wrote about the PPP 20 years ago: you can plug your pseudocode into AI code generation tools and get the best of both worlds. You get to design the routine and think through all the problems likely to be encountered to keep the AI on track, and the computer gets to do the tedious job of turning it into real code. You can prompt the AI to keep all the pseudocode as comments to make your job reviewing that what it’s produced matches the intent much easier.
You can then go one more step up the meta ladder and prompt the AI to first write the pseudocode! You can then review the high level pseudocode, iterate on it (generate a couple variations if needed), and make sure it meets all the design criteria. You’d better understand how the pseudocode will work before generating code with it or else you’re going to really be lost when reviewing the code.
The complexity level of your function and how much time you spend on the pseudocode will determine your results but I expect it to be a very useful technique as code generation AIs improve and as you become more proficient at designing in pseudocode.
Conclusion & Bonus Uses
The Pseudocode Programming Process is a powerful technique for designing classes, functions and components at a high level before spending the time translating into working code. It lets you find problems early and iterate to fix them while they’re the easiest to fix, and when you’ve finished the process you end up with well documented code.
The process is similar to the outlining technique you might have used for writing a term paper and I even used it to write this post!
Another good time to pull it out of your back pocket is in your next coding interview. Ask the interviewer about the design conditions to make sure you understand the problem, then start writing the pseudocode and see if you can get any feedback on it from the interviewer before translating it into the working code. If any candidate I was interviewing did this I would certainly be impressed.
I’m sure there’s even more applications I’m missing where the PPP can be applied so let me know in the comments if you think of any!
Want the inside scoop?
Sign up and be the first to see new posts
No spam, just the inside scoop and $10 off any photo print!